Create Aliases For Long Scripts with npm-run-scripts
Sometimes you want to run a script in the console, but sometimes it’s too flippin long. It could be a script to lint, to build, to test, to deploy that might include additional variables.
For example, to build my React app, I have to run this command in the terminal:
HEAD=\"$(git rev-parse HEAD)\" webpack --env=development --mode production --config webpack.config.js --output-path dist
That is a pretty long line! Do I have to type that many characters in the terminal every time I want to build my app? I don’t think I could remember all of that.
Arghh…what should I do? Should I just copy and paste it somewhere, and then when I need it, I’ll copy it back into the terminal? NO!! There must be a better way!
npm-run-scripts
You can create aliases by adding them to the "scripts"
object of your package.json
file. The keys in the "script"
object (left of the colon) are used as the script alias and the value (right of the colon) is what will be executed when the script alias is used.
{
"name": "my-custom-app",
"version": "1.0.0",
"description": "My cool custom app",
"author": "Jennifer Pham",
"repository": "https://github.com/jenniferpham/my-custom-app",
"license": "UNLICENSED",
"scripts": {
"build": "HEAD=\"$(git rev-parse HEAD)\" webpack --env=development --mode production --config webpack.config.js --output-path dist"
},
"dependencies": {
...
},
"devDependencies": {
...
}
}
After you add "scripts"
object to your package.json
, you can run alias commands in the terminal.
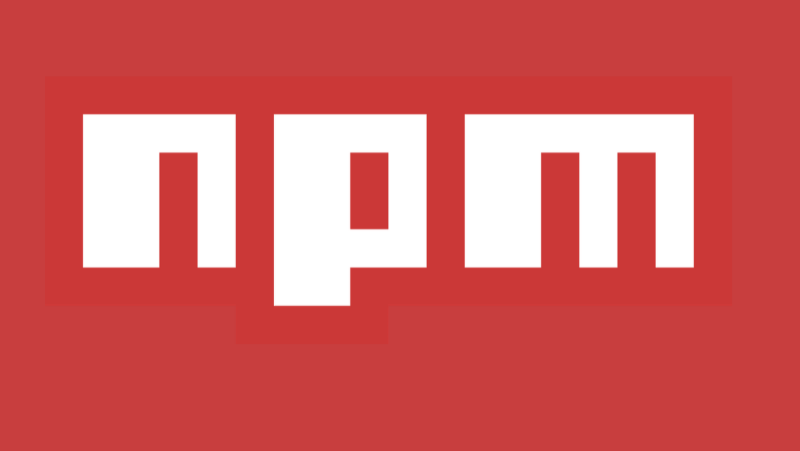
If you’re using npm
, then run this command in the terminal
npm run build
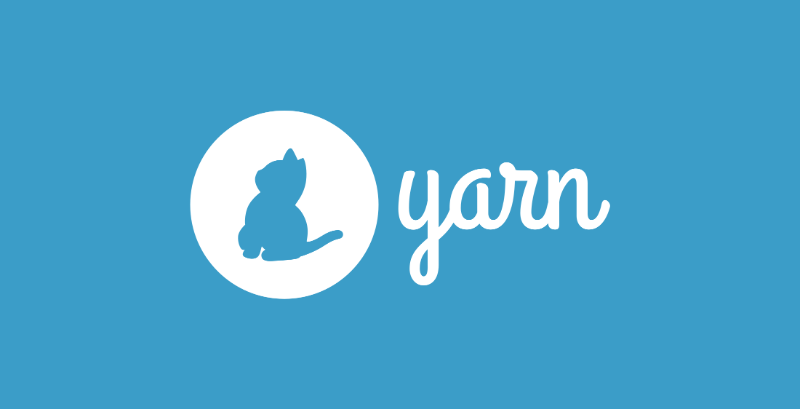
or if you’re using yarn
, then run this command
yarn run build
If you’re using npm | If you’re using yarn |
npm run [alias-command] | yarn run [alias-command] |
Once you press enter, it will automatically run this longer command
HEAD=\"$(git rev-parse HEAD)\" webpack --env=development --mode production --config webpack.config.js --output-path dist
So now you know. Go crazy with your script shortcuts. I know I have!
Other script aliases that I’ve used
{
"name": "my-custom-app",
"version": "1.0.0",
"description": "My cool custom app",
"author": "Jennifer Pham",
"repository": "https://github.com/jenniferpham/my-custom-app",
"license": "UNLICENSED",
"scripts": {
"analyze-prod-bundle": "ANALYZE=1 yarn build:production",
"dev": "HEAD=\"$(git rev-parse HEAD)\" NODE_ENV=development webpack-dev-server",
"dev:open": "yarn dev --open",
"prebuild": "yarn clean",
"build": "HEAD=\"$(git rev-parse HEAD)\" webpack --env=development --mode production --config webpack.config.js --output-path dist",
"build:production": "HEAD=\"$(git rev-parse HEAD)\" webpack --env=production --mode production --config webpack.config.js --output-path dist-production",
"clean": "rimraf dist && rimraf .cache",
"lint:css": "stylelint 'src/**/*.{ts,tsx}'",
"lint": "tslint -c tslint.json --format stylish 'src/**/*.{ts,tsx}'",
"precommit": "lint-staged",
"test": "TZ=America/Los_Angeles jest --maxWorkers=6 -c='./jest.config.js'",
"test:watch": "yarn test --watch"
},
"dependencies": {
...
},
"devDependencies": {
...
}
}
What script aliases have you written?
I know a lot of apps have a lot of different needs, and people come up with a lot of great stuff. I’m always interested in automating tedious tasks. I’d love to get good ideas on any useful script shortcuts that other people have created. If you have written a script shortcut that you’re proud of and saved yourself (and others) time, please share in the comment below. Let’s all learn from each other.
Very clear and concise article Jen !
Good info to keep handy! Saves a lot of time and makes things frustration-free!